OpenCV: Hello, World!
Hello World seems to be the simplest program program that a novice program can run and compile. However, in OpenCV sure you can copy the Hello, World! application but compiling and running it is a feat itself which I am going to simplify in this tutorial.
First things first, let’s clear things up with the source code in a step-by-step approach. It is worth mentioning that I will be using C++ because it is more tricky and less resources are available so I thought it could be of a more value.
Step 1: C++ Starter Template
#include <iostream>
using namespace std;
int main() {
cout << "Everything works here" << endl;
return 0;
}
I will save this piece of code in a file, let’s say, app.cpp and we can compile, run, and delte the object using below commands respectively (I often delete object to perserve productivity of my files and sources).
g++ app.cpp -o app
./app
rm app
Step 2: Load OpenCV
To load OpenCV with C++ the most convenient way is to load linker files while compiling applications. Some people tend to provide these files manually which can be significantly tedious that is why I always advise using pkg-config.
g++ app.cpp -o app $(pkg-config --cflags --libs opencv4)
./app
rm app
If you for some reason had not had pkg-config installed on your machine take some time and install and configure it with OpenCV. Believe me it would save you a great deal of time while working on your projects.
You have to be able to run pkg-config —cflags —libs opencv4 separately and get a result like:
-I/usr/local/include/opencv4 -L/usr/local/lib -lopencv_gapi -lopencv_highgui -lopencv_ml -lopencv_objdetect -lopencv_photo -lopencv_stitching -lopencv_video -lopencv_calib3d -lopencv_features2d -lopencv_dnn -lopencv_flann -lopencv_videoio -lopencv_imgcodecs -lopencv_imgproc -lopencv_core
At this point, although we loaded OpenCV’s linkers we have not used responsible general header file to load in our source file in order to do that we will modify our application like following.
#include <iostream>
#include <opencv2/opencv.hpp>
using namespace std;
using namespace cv;
int main() {
cout << "Everything works here" << endl;
return 0;
}
If above application compiles and run successfuly, you are good to go with the rest of tutorial, otherwise you have to take some time to rectify the behaviour of g++ to be able to compile OpenCV applications.
Step 3: Hello, World OpenCV!
#include <iostream>
#include <opencv2/opencv.hpp>
using namespace std;
using namespace cv;
int main() {
Mat image(400, 400, CV_8UC1);
putText(
image,
"Hello, OpenCV!",
Point(75, 200),
FONT_HERSHEY_SIMPLEX,
1,
Scalar(255,255,255),
2
);
namedWindow("Hello");
imshow("Hello", image);
waitKey(0);
return 0;
}
Finally, you have to face something like this.
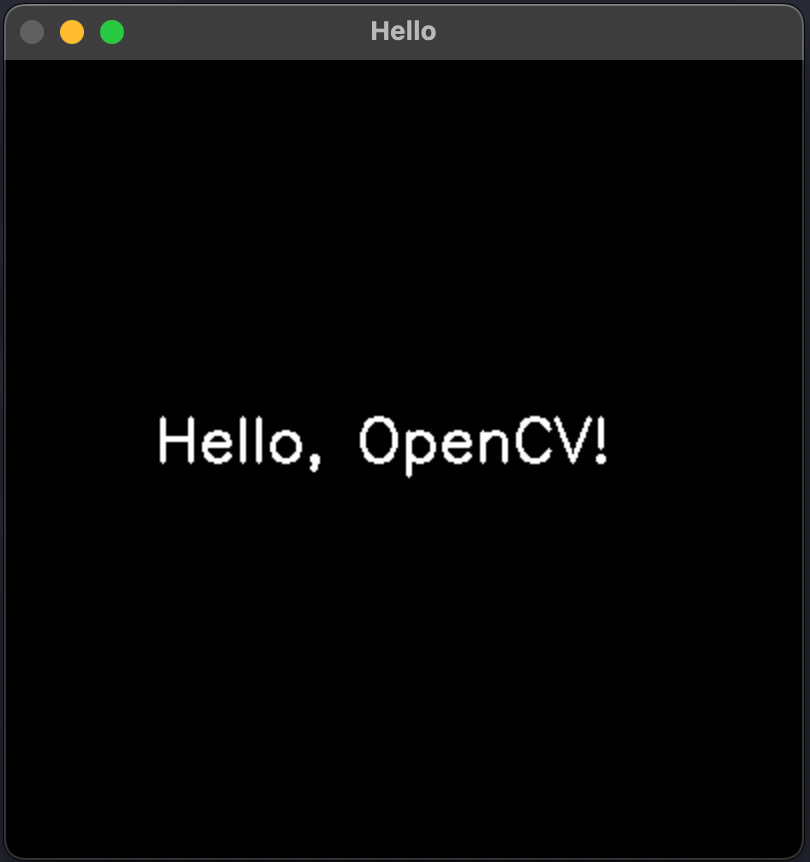